
A frequent customer asks us how we can implement a simple yet smart solution to charge an electric car only at the cheapest electricity tariff, using Nordpool electricity exchange prices.
The answer is very simple. This solution can be used not only for charging electric cars, but also for heating water or other needs.
The operating principle is quite simple. The smart relay controls the contact, switches on/off according to the script, and has to control the CP contact at the charging station.
Script
let CONFIG = {
api_endpoint: "https://dashboard.elering.ee/api/nps/price/lt/current",
switchId: 0, // Jūsų rėlės ID. Jeigu vieno kontakto, bus 0. Jeigu kelių, pagal numerį
price_limit: 200, // EUR/MWh. Svarbu, kaina be PVM.
update_time: 60000, // 1 minutė. Kas kiek laiko tikrinsime kainas ?
reverse_switching: false // Įjungia rėlę, jeigu atitinka Jūsų kainą, jeigu ne, išjungia.
};
let current_price = null;
let last_hour = null;
let last_price = null;
let state = null;
function getCurrentPrice() {
Shelly.call(
"http.get",
{
url: CONFIG.api_endpoint,
},
function (response, error_code, error_message) {
if (error_code !== 0) {
print(error_message);
return;
}
current_price = JSON.parse(response.body).data[0]["price"];
print("Updated current price!");
}
);
}
function changeSwitchState(state) {
let state = state;
if(state === false) {
print("Switching off!");
} else if(state === true) {
print("Switching on!");
} else {
print("Unknown state");
}
Shelly.call(
"Switch.Set",
{
id: CONFIG.switchId,
on: state,
},
function (response, error_code, error_message) {
if (error_code !== 0) {
print(error_message);
return;
}
}
);
}
Timer.set(CONFIG.update_time, true, function (userdata) {
Shelly.call("Sys.GetStatus", {}, function (resp, error_code, error_message) {
if (error_code !== 0) {
print(error_message);
return;
} else {
let hour = resp.time[0] + resp.time[1];
//atnaujiname kainas
if (last_hour !== hour) {
print("update hour");
last_hour = hour;
getCurrentPrice();
}
//Tinkriname ar nustatyta kaina
if (current_price !== null) {
//Standartinis ijungimas. Išjungiame, jeigu virisijame limita
if(CONFIG.reverse_switching === false) {
if (current_price >= CONFIG.price_limit) {
//isjungiame, jeigu virs limito
changeSwitchState(false);
} else {
//ijungiame, jeigu tenkina salygas limito
changeSwitchState(true);
}
}
//reversinis variantas.
if(CONFIG.reverse_switching === true) {
if (current_price >= CONFIG.price_limit) {
//ijungiame, jeigu netenkina limito salygos
changeSwitchState(true);
} else {
//isjungiame, jeigu tenkina limito salygas
changeSwitchState(false);
}
}
} else {
print("Current price is null. Waiting for price update!");
}
print(current_price);
}
});
});
You will need a smart relay, we recommend the Shelly smart relay. After connecting directly to the relay, under Scripts, copy the script itself, and don’t forget to run the script after saving it.
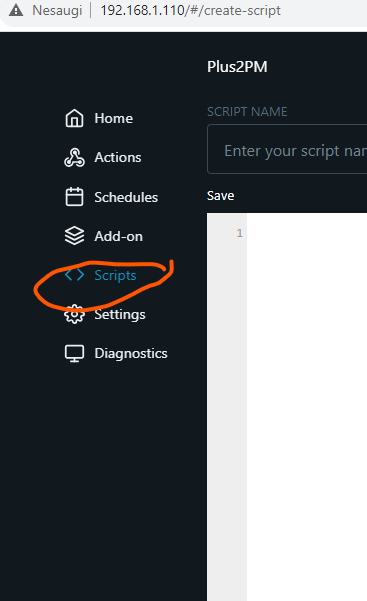
-
10.2kW SE kit hybrid inverter + TrinaSolar 425W Black
Original price was: €6,715.50.€5,747.50Current price is: €5,747.50. -
10.4kW ground-mounted solar power plant kit + installation
Original price was: €11,024.31.€10,618.96Current price is: €10,618.96. -
10kW Premium Solar Power Plant + Wallbox Pulsar Charging Station
Original price was: €12,100.00.€10,890.00Current price is: €10,890.00. -
1x22kW charging station rental
Original price was: €90.75.€66.55Current price is: €66.55. -
ABB B21 112-100 Energy Meter, Modular, DIN Rail, 1 Phase, 65 A, 220 to 240 Vac, Class B, Pulse Output, RS485
€194.81 -
ABB B23 112-100, Energy Meter, Modular, DIN-rail mounted 3 phase, 65 A
€204.49 -
ABB Terra AC 11kW + Power Controller B23 112-100 Kit for ENA support
Original price was: €1,034.55.€900.24Current price is: €900.24. -
ABB Terra AC Type2 socket
€551.76 – €671.55 -
ABB Terra AC with Type 2 cable
€643.72 – €744.15